1. int.getline()함수를ㅇ 이용하여 키보드로부터 한 라인을 읽고 'a'가 몇개인지 출력하는 프로그램을 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <istream>
using namespace std;
int main() {
int ch;
int count{};
cout << "a를 입력하세요 >> ";
while((ch = cin.get()) != EOF) {
if (ch == '\n')
break;
else if(ch == 'a')
count++;
}
cout << count;
}
2. istream& get(char& ch) 함수를 이용하여 한 라인을 읽고 빈칸(' ')이 몇 개인지 출력하는 프로그램을 작성하라.
[결과]

[소스 코드]
#include <iostream>
using namespace std;
int main() {
cout << "빈칸의 개수를 출력하는 프로그램입니다. 문자열을 입력하세요 >> ";
char ch;
int count{};
while (true) {
cin.get(ch);
if (cin.eof())
break;
else if (ch == ' ')
count++;
else if (ch == '\n')
break;
}
cout << count;
}
3. 한 줄에 '영어문장 ; 한글문장' 형식으로 키 입력될 때 cin.ignore()을 이용하여 ';' 이후에 입력된 문자열을 화면에 출력하는 프로그램을 작성하라. 아래에서 ^z 키는 입력 종료를 나타내는 키이며, cin.get()은 EOF를 리턴한다.
[결과]

[소스 코드]
#include <iostream>
using namespace std;
int main() {
char ch;
cout << "문자열을 입력해주세요, ;가 기준입니다. " << endl;
cin.ignore(100, ';');
while ((ch = cin.get()) != EOF) {
cout.put(ch);
if (ch == '\n')
cin.ignore(100, ';');
}
}
4. 한 줄에 '영어문장;한글문자' 형식으로 키 입력될 때 cin.ignore()를 이용하여 ';'이전에 입력된 문자열만 출력하는 프로그램을 작성하라. 아래에서 ^z 키는 입력 종료를 나타내는 키이며, cin.get()은 EOF를 리턴한다.
[결과]

[소스 코드]
#include <iostream>
using namespace std;
int main() {
char ch;
cout << "문자열을 입력해주세요, ;가 기준입니다. " << endl;
while ((ch = cin.get()) != EOF) {
if (ch == ';') {
cout.put('\n');
cin.ignore(100, '\n');
}
else
cout.put(ch);
}
}
5. 다음 프로그램은 예제 11-3의 코드이다. 아래 코드에서 char[] 대신 string을 이용하여 문자열을 다루도ㅓ록 프로그램을 재작성하라.
[결과]

[소스 코드]
#include <iostream>
#include<string>
using namespace std;
int main() {
string cmd;
cout << "getline()으로 문자열을 읽습니다. " << endl;
while (true) {
cout << "종료하려면 exit를 입력하세요 >> ";
getline(cin, cmd);
if (cmd == "exit") {
cout << "프로그램을 종료합니다. --- ";
return 0;
}
}
}
6. 다음과 같이 정수, 제곱, 제곱근의 값을 형식에 맞추어 출력하는 프로그램을 작성ㅎ라라. 필드의 간격은 총 15칸이고 제곱근의 유효 숫자 거리는 총 3자리로 한다. 빈칸은 모두 underline(_) 문자로 한다.
[결과]
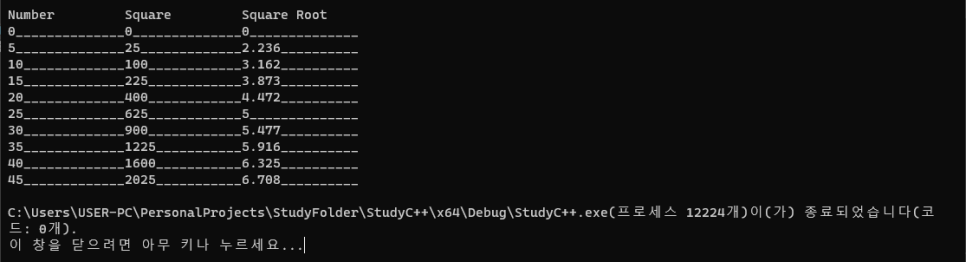
[소스 코드]
#include <iostream>
#include <iomanip>
#include<cmath>
using namespace std;
int main() {
cout.setf(ios::left);
cout << setw(15) << "Number";
cout << setw(15) << "Square";
cout << setw(15) << "Square Root";
cout << endl;
for (int i = 0; i <= 45; i += 5)
{
cout << setw(15) << setfill('_') << i;
cout << setw(15) << setfill('_') << pow(i, 2);
double j = sqrt(i);
cout.precision(4);
cout << setw(15) << setfill('_') << j;
cout << endl;
}
}
7. 0에서 127까지 ASKII 코드와 해당 문자를 다음과 같이 출력하ㅣ는 프로그램을 작성하라. 화면에 출력가능하지 않는 ASKII 코드는 '.'으로 출력하라.
[결과]
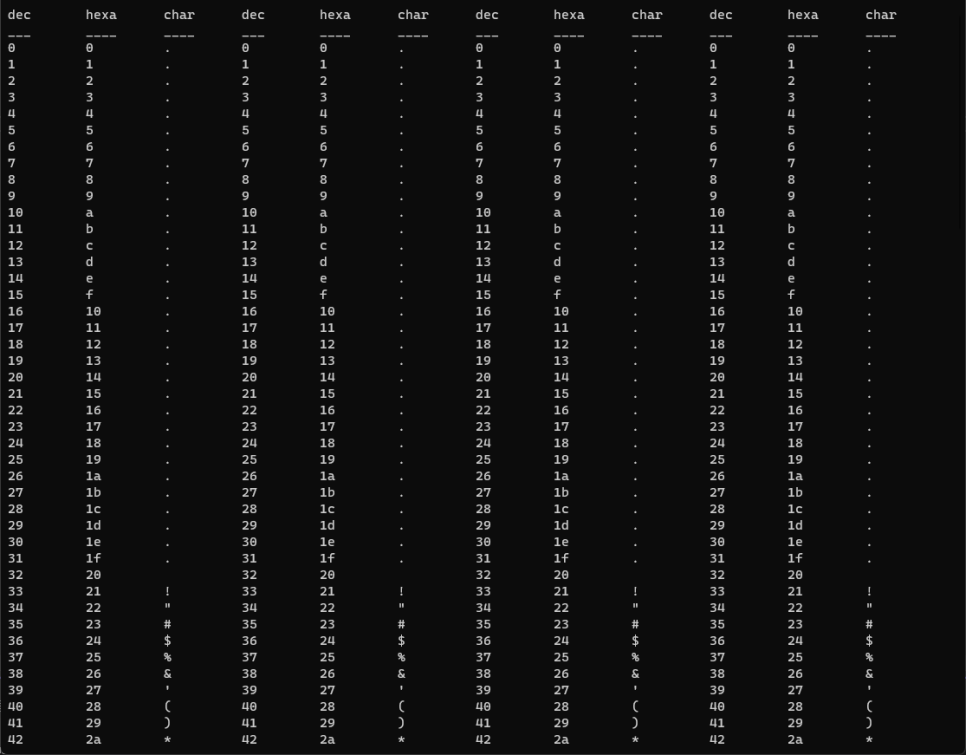
[소스 코드]
#include <iostream>
#include <iomanip>
#include <cctype>
#include<cmath>
using namespace std;
int main() {
cout.setf(ios::left);
for (int i = 0; i < 4; i++) {
cout << setw(10) << "dec";
cout << setw(10) << "hexa";
cout << setw(10) << "char";
}
cout << endl;
for (int i = 0; i < 4; i++) {
cout << setw(10) << "___";
cout << setw(10) << "____";
cout << setw(10) << "____";
}
cout << endl;
char c;
for (int i = 0; i < 128; i++){
for (int j = 0; j < 4; j++) {
cout << setw(10) << setfill(' ') << dec << i;
cout << setw(10) << setfill(' ') << hex << i;
if (isprint(i))
c = (char)i;
else c = '.';
cout << setw(10) << setfill(' ') << c;
}
cout << endl;
}
}
8. Circle 클래스는 다음과 같다. . . . Circle 클래스의 객체를 입출력하는 다음 코드와 실행 결과를 참조하여 <<, >> 여연산자를 작성하고, Circle 클래스를 수정하는 등 프로그램을 완성하라.
[결과]

[소스 코드]
#include <iostream>
#include <iomanip>
#include <cctype>
#include<cmath>
#include <string>
using namespace std;
class Circle {
string name;
int radius;
public:
Circle(int radius = 1, string name = " ") {
this->radius = radius; this->name = name;
}
friend istream& operator >> (istream& stream, Circle &p);
friend ostream& operator << (ostream& stream, Circle &p);
};
istream &operator >> (istream &stream, Circle &p) {
cout << "반지름 > ";
cin >> p.radius;
cin.ignore();
cout << "이름 > ";
getline(cin, p.name);
return stream;
}
ostream& operator << (ostream& stream, Circle &p) {
cout << "반지름이 " << p.radius << "인 " << p.name;
return stream;
}
int main() {
Circle d, w;
cin >> d >> w;
cout << d << w << endl;
}
9. 다음은 Phone 클래스이다. . . Phone 클래스의 객체를 입출력하는 아래 코드와 실행 결과를 참조하여 <<, >> 연산자를 작성하고 Phone 클래스를 수정하는 등 프로그램을 완성하라.
[결과]
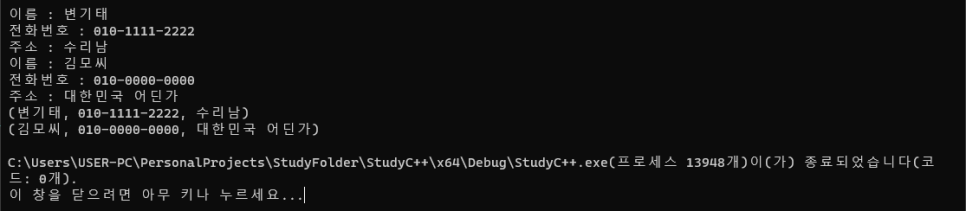
[소스 코드]
#include <iostream>
#include <iomanip>
#include <cctype>
#include<cmath>
#include <string>
using namespace std;
class Phone {
string name;
string telnum;
string address;
public:
Phone(string name = " ", string telnum = " ", string address = " ") {
this->name = name;
this->telnum = telnum;
this->address = address;
}
friend istream& operator >> (istream& stream, Phone& p);
friend ostream& operator << (ostream& stream, Phone& p);
};
istream &operator >> (istream &stream, Phone &p) {
cout << "이름 : ";
cin >> p.name;
cout << "전화번호 : ";
cin >> p.telnum;
cin.ignore();
cout << "주소 : ";
getline(cin, p.address);
return stream;
}
ostream& operator << (ostream& stream, Phone &p) {
cout << "(" << p.name << ", " << p.telnum << ", " << p.address << ")";
return stream;
}
int main() {
Phone girl, boy;
cin >> girl >> boy;
cout << girl << endl << boy << endl;
}
10. 다음은 프로그램과 실행 결과를 보여준다 prompt 조작자를 작성하여 프로그램을 완성하라.
[결과]

[소스 코드]
#include <iostream>
#include <iomanip>
#include <cctype>
#include<cmath>
#include <string>
using namespace std;
istream &prompt(istream& ins) {
cout << "암호 ? ";
return ins;
}
int main() {
string password;
while (true) {
cin >> prompt >> password;
if (password == "C++"){
cout << "Login success!" << endl;
break;
}
else
cout << "Login failed!" << endl;
}
}
11. 다음은 프로그램과 실행 결과를 보여준다. pos 조작자를 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <iomanip>
#include <cctype>
#include<cmath>
#include <string>
using namespace std;
istream& pos(istream& ins) {
cout << "위치는 ? ";
return ins;
}
int main() {
int x, y;
cin >> pos >> x;
cin >> pos >> y;
cout << x << ',' << y << endl;
}
12. 커피 자판기 시뮬레이터를 C++로 작성해보자. 실행 사례는 다음과 같다. 자판기는 보통 커피, 설탕 커피, 블랙 커피의 3종류만 판매한다. 단순화를 위해 실행 사례에는 총 3인분의 재료만 가지도록 하였다. 커피 메뉴에 따라 필요한 재료들이 하나씩 없어진다. 객체 지향 구조ㅓ에 따라 필요한 클래스를 작성하여 프로그램을 완성하라.
[결과]
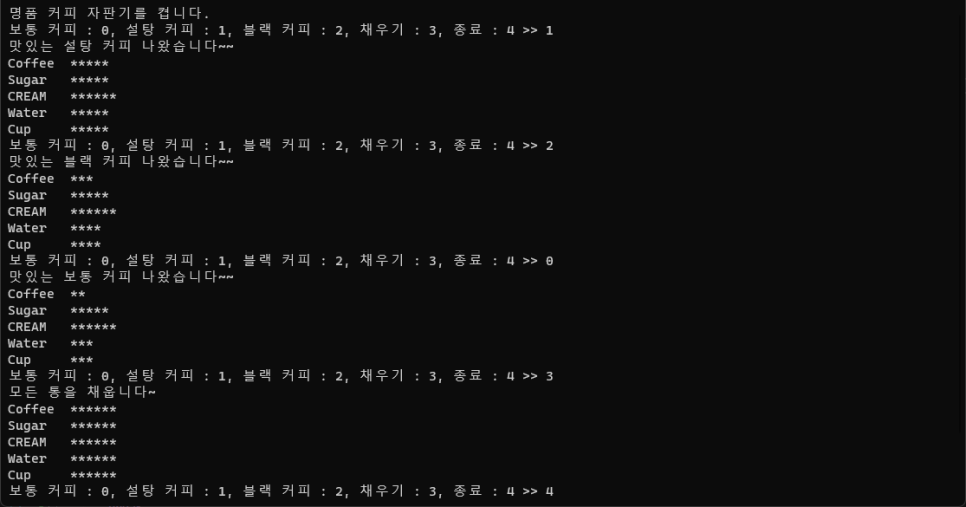
[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class CoffeeMachine {
protected:
int coffeeLeft{};
int coffeeList[5];
public:
CoffeeMachine();
void show();
bool inputChoice();
void refill();
};
CoffeeMachine::CoffeeMachine() {
cout << "명품 커피 자판기를 켭니다." << endl;
for (int i = 0; i < 5; i++)
coffeeList[i] = 5;
}
void CoffeeMachine::refill() {
cout << "모든 통을 채웁니다~" << endl;
for (int i = 0; i < 5; i++)
coffeeList[i] = 5;
}
bool CoffeeMachine::inputChoice() {
int choice;
cin >> choice;
switch (choice) {
case 0:// 커피
cout << "맛있는 보통 커피 나왔습니다~~ " << endl;
coffeeList[0]--;
coffeeList[3]--;
coffeeList[4]--;
break;
case 1:// 설탕
cout << "맛있는 설탕 커피 나왔습니다~~ " << endl;
coffeeList[0]--;
coffeeList[1]--;
coffeeList[3]--;
coffeeList[4]--;
break;
case 2: // 블랙
cout << "맛있는 블랙 커피 나왔습니다~~ " << endl;
coffeeList[0] -= 2;
coffeeList[3]--;
coffeeList[4]--;
break;
case 3: // 모두 채우기
refill();
break;
case 4: // 종료
return false;
break;
default:
cout << "입력이 잘못되었습니다." << endl;
break;
}
}
void CoffeeMachine::show() {
int kind{ };
cout << "Coffee" << "\t";
for (int i = 0; i <= coffeeList[kind]; i++) {
cout << "*";
}
kind++;
cout << endl;
cout << "Sugar" << "\t";
for (int i = 0; i <= coffeeList[kind]; i++) {
cout << "*";
}
cout << endl;
kind++;
cout << "CREAM" << "\t";
for (int i = 0; i <= coffeeList[kind]; i++) {
cout << "*";
}
cout << endl;
kind++;
cout << "Water" << "\t";
for (int i = 0; i <= coffeeList[kind]; i++) {
cout << "*";
}
cout << endl;
kind++;
cout << "Cup" << "\t";
for (int i = 0; i <= coffeeList[kind]; i++) {
cout << "*";
}
cout << endl;
}
static void run() {
CoffeeMachine coffeMachine;
while (true) {
cout << "보통 커피 : 0, 설탕 커피 : 1, 블랙 커피 : 2, 채우기 : 3, 종료 : 4 >> ";
if (!coffeMachine.inputChoice())
break;
coffeMachine.show();
}
}
int main() {
run();
}
책에서 나오는 힌트나 조건들의 일부는 저작자 존중을 위해 일부러 작성하지 않았습니다.
개인 공부용으로 올리는 만큼 풀이에 대한 지적 감사하겠습니다.
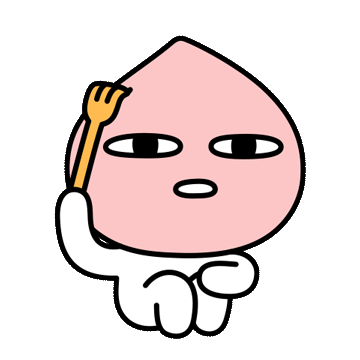
'C-C++ > 명품 C++ Programming' 카테고리의 다른 글
[(개정판) 명품 C++ programming] 10장 실습 문제 (0) | 2023.01.07 |
---|---|
[(개정판) 명품 C++ programming] 9장 실습 문제 (0) | 2023.01.07 |
[(개정판) 명품 C++ programming] 8장 실습 문제 (2) | 2023.01.07 |
[(개정판) 명품 C++ programming] 7장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 6장 실습 문제 (0) | 2023.01.06 |