※문제 1 ~ 4까지 사용될 Book 클래스는 다음과 같다.
class Book
{
string title;
int price, pages;
public:
Book(string title = " ", int price = 0, int pages = 0)
{
this->title = title;
this->price = price;
this->pages = pages;
}
void show()
{
cout << title << ' ' << price << "원 " << pages << "페이지" << endl;
}
string getTitle()
{
return title;
}
};
1. Book 객체에 대해 다음 연산을 하고자 한다.
[결과]

1-1. +=, -= 연산자 함수를 Book 클래스의 멤버 함수로 구현하라.
#include <iostream>
using namespace std;
class Book
{
string title;
int price, pages;
public:
Book(string title = " ", int price = 0, int pages = 0)
{
this->title = title;
this->price = price;
this->pages = pages;
}
Book &operator += (int x)
{
price = price + x;
return *this;
}
Book& operator -= (int x)
{
price = price - x;
return *this;
}
void show()
{
cout << title << ' ' << price << "원 " << pages << "페이지" << endl;
}
string getTitle()
{
return title;
}
};
int main()
{
Book a("청춘", 20000, 300), b("미래", 30000, 500);
a += 500;
b -= 500;
a.show();
b.show();
}
1-2. +=, -= 연산자 함수를 Book 클래스 외부 함수로 구현하라.
#include <iostream>
using namespace std;
class Book
{
string title;
int price, pages;
public:
Book(string title = " ", int price = 0, int pages = 0)
{
this->title = title;
this->price = price;
this->pages = pages;
}
friend Book& operator += (Book &op, int x);
friend Book& operator -= (Book &op, int x);
void show()
{
cout << title << ' ' << price << "원 " << pages << "페이지" << endl;
}
string getTitle()
{
return title;
}
};
Book& operator += (Book& op, int x)
{
op.price = op.price + x;
return op;
}
Book &operator -= (Book& op, int x)
{
op.price = op.price - x;
return op;
}
int main()
{
Book a("청춘", 20000, 300), b("미래", 30000, 500);
a += 500;
b -= 500;
a.show();
b.show();
}
2. Book 객체를 활용하는 사례이다.
[결과]

2-1. 세 개의 == 연산자 함수를 가진 Book 클래스를 작성하라.
#include <iostream>
using namespace std;
class Book
{
string title;
int price, pages;
public:
Book(string title = " ", int price = 0, int pages = 0)
{
this->title = title;
this->price = price;
this->pages = pages;
}
bool operator == (int price)
{
if (this->price == price)
return true;
else return false;
}
bool operator == (string title)
{
if (this->title == title)
return true;
else return false;
}
bool operator == (Book op2)
{
if (this->title == op2.title && this->price == op2.price && this->pages == op2.pages)
return true;
else return false;
}
void show()
{
cout << title << ' ' << price << "원 " << pages << "페이지" << endl;
}
string getTitle()
{
return title;
}
};
int main()
{
Book a("명품 C++", 30000, 500), b("고품 C++", 30000, 500);
if (a == 30000) cout << "정가 30000원" << endl;
if (a == "명품 C++") cout << "명픔 C++입니다." << endl;
if (a == b) cout << "두 책이 같은 책입니다. " << endl;
}
2-2. 세 개의 == 연산자를 프렌드 함수로 작성하라.
#include <iostream>
using namespace std;
class Book
{
string title;
int price, pages;
public:
Book(string title = " ", int price = 0, int pages = 0)
{
this->title = title;
this->price = price;
this->pages = pages;
}
bool operator == (int price)
{
if (this->price == price)
return true;
else
return false;
}
bool operator == (string title)
{
if (this->title == title)
return true;
else
return false;
}
bool operator == (Book op2)
{
if (this->title == op2.title && this->price == op2.price && this->pages == op2.pages)
return true;
else
return false;
}
void show()
{
cout << title << ' ' << price << "원 " << pages << "페이지" << endl;
}
string getTitle()
{
return title;
}
};
int main()
{
Book a("명품 C++", 30000, 500), b("고품 C++", 30000, 500);
if (a == 30000) cout << "정가 30000원" << endl;
if (a == "명품 C++") cout << "명픔 C++입니다." << endl;
if (a == b) cout << "두 책이 같은 책입니다. " << endl;
}
3. 다음 연산을 통해 공짜 책인지 판별하도록 ! 연산자를 작성하라.
[결과]

[! 연산자와 메인 함수]
bool operator !()
{
if (price == 0)
return true;
else
return false;
}
. . .
int main()
{
Book book("벼룩시장", 0, 50);
if (!book)
cout << "공짜다. " << endl;
}
4. 다음 연산을 통해 책의 제목을 사전 순으로 비교하고자 한다. < 연산자를 활용하라.
[결과]

[< 연산자와 메인 함수]
friend bool operator < (string name, Book op);
. . .
bool operator < (string name, Book op)
{
if (name < op.title)
return true;
else
return false;
}
int main()
{
Book a("청춘", 20000, 300);
string b;
cout << "책 이름을 입력하세요. > ";
getline(cin, b);
if (b < a)
cout << a.getTitle() << "이 " << b << "보다 뒤에 있구나!" << endl;
}
5. 다음 main()에서 Color 클래스는 3요소(빨강, 초록, 파랑)로 하나의 색을 나타내는 클래스이다.(4장 실습문제 1번 참고). + 연산자로 색을 더하고, == 연산자로 색을 비교하고자 한다. 실행 결과를 참고하여 Color 클래스와 연산자, 그리고 프로그래밍을 완성하라.
[결과]

5-1. + 와 == 연산자를 Color 클래스의 멤버 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Color
{
int red, green, blue;
public:
Color(int red = 0, int green = 0, int blue = 0)
{
this->red = red;
this->green = green;
this->blue = blue;
}
Color operator + (Color op2)
{
Color tmp;
tmp.red = this->red + op2.red;
tmp.green = this->green + op2.green;
tmp.blue = this->blue + op2.blue;
return tmp;
}
bool operator == (Color fu)
{
if (red == fu.red && green == fu.green && blue == fu.blue)
return true;
else
return false;
}
void show();
};
void Color::show()
{
cout << red << ' ' << green << ' ' << blue << endl;
}
int main()
{
Color red(255, 0, 0), blue(0, 0, 255), c;
c = red + blue;
c.show();
Color fuchsia(255, 0, 255);
if (c == fuchsia)
cout << "보라색 맞음";
else
cout << "보라색 아님";
}
5-2. + 와 == 연산자를 Color 클래스의 프렌드 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Color
{
int red, green, blue;
public:
Color(int red = 0, int green = 0, int blue = 0)
{
this->red = red;
this->green = green;
this->blue = blue;
}
friend Color operator + (Color op1, Color op2);
friend bool operator == (Color op3, Color fu)
{
if (op3.red == fu.red && op3.green == fu.green && op3.blue == fu.blue)
return true;
else
return false;
}
void show();
};
Color operator + (Color op1, Color op2)
{
Color tmp;
tmp.red = op1.red + op2.red;
tmp.green = op1.green + op2.green;
tmp.blue = op1.blue + op2.blue;
return tmp;
}
void Color::show()
{
cout << red << ' ' << green << ' ' << blue << endl;
}
int main()
{
Color red(255, 0, 0), blue(0, 0, 255), c;
c = red + blue;
c.show();
Color fuchsia(255, 0, 255);
if (c == fuchsia)
cout << "보라색 맞음";
else
cout << "보라색 아님";
}
6. 2차원 행렬이 가능하도록 Matrix 클래스를 작성하고, show() 멤버 함수와 다음 연산이 가능하도록 연산자를 모두 구현하라.
[결과]

6-1. 연산자 함수를 Matrix의 멤버 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Matrix
{
int first, second, third, fourth;
public:
Matrix(int first = 0, int second = 0, int third = 0, int fourth = 0)
{
this->first = first;
this->second = second;
this->third = third;
this->fourth = fourth;
}
Matrix operator + (Matrix op2)
{
Matrix tmp;
tmp.first = this->first + op2.first;
tmp.second = this->second + op2.second;
tmp.third = this->third + op2.third;
tmp.fourth = this->fourth + op2.fourth;
return tmp;
}
Matrix& operator += (Matrix op2)
{
this->first += op2.first;
this->second += op2.second;
this->third += op2.third;
this->fourth += op2.fourth;
return *this;
}
bool operator == (Matrix op3)
{
if (this->first == op3.first && this->second == op3.second && this->third == op3.third && this->fourth == op3.fourth)
return true;
else
return false;
}
void show()
{
cout << "Matrix = {" << first << ' ' << second << ' ' << third << ' ' << fourth << "} \n";
}
};
int main()
{
Matrix a(1, 2, 3, 4), b(2, 3, 4, 5), c;
c = a + b;
a += b;
a.show(); b.show(); c.show();
if (a == c)
cout << "a and c are the smae" << endl;
}
6-2. 연산자 함수를 Matrix의 프렌드 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Matrix
{
int first, second, third, fourth;
public:
Matrix(int first = 0, int second = 0, int third = 0, int fourth = 0)
{
this->first = first;
this->second = second;
this->third = third;
this->fourth = fourth;
}
friend Matrix operator + (Matrix op1, Matrix op2);
friend Matrix& operator += (Matrix &op1, Matrix op2);
friend bool operator == (Matrix op1, Matrix op3);
void show()
{
cout << "Matrix = {" << first << ' ' << second << ' ' << third << ' ' << fourth << "} \n";
}
};
Matrix operator + (Matrix op1, Matrix op2)
{
Matrix tmp;
tmp.first = op1.first + op2.first;
tmp.second = op1.second + op2.second;
tmp.third = op1.third + op2.third;
tmp.fourth = op1.fourth + op2.fourth;
return tmp;
}
Matrix& operator += (Matrix &op1, Matrix op2)
{
op1.first += op2.first;
op1.second += op2.second;
op1.third += op2.third;
op1.fourth += op2.fourth;
return op1;
}
bool operator == (Matrix op1, Matrix op3)
{
if (op1.first == op3.first && op1.second == op3.second && op1.third == op3.third && op1.fourth == op3.fourth)
return true;
else
return false;
}
int main()
{
Matrix a(1, 2, 3, 4), b(2, 3, 4, 5), c;
c = a + b;
a += b;
a.show(); b.show(); c.show();
if (a == c)
cout << "a and c are the smae" << endl;
}
7. 2차원 행렬을 추상화한 Matrix 클래스를 활용하는 다음 코드가 있다.
[결과]

7-1. << , >> 연산자 함수를 Matrix의 멤버 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Matrix
{
int a, b, c, d;
public:
Matrix(int a = 0, int b = 0, int c = 0, int d = 0)
{
this->a = a;
this->b = b;
this->c = c;
this->d = d;
}
Matrix operator >> (int arr[])
{
int* p = arr;
p[0] = this->a;
p[1] = this->b;
p[2] = this->c;
p[3] = this->d;
return *p;
}
Matrix& operator << (int arr[])
{
this->a = arr[0];
this->b = arr[1];
this->c = arr[2];
this->d = arr[3];
return *this;
}
void show()
{
cout << "Matrix = {" << a << ' ' << b << ' ' << c << ' ' << d << "} \n";
}
};
int main()
{
Matrix a(4, 3, 2, 1), b;
int x[4], y[4] = { 1, 2, 3, 4 };
a >> x;
b << y;
for (int i = 0; i < 4; i++)
cout << x[i] << ' ';
cout << endl;
b.show();
}
7-2. << , >> 연산자 함수를 Matrix의 프렌드 함수로 구현하라.
#include <iostream>
#include <string>
using namespace std;
class Matrix
{
int a, b, c, d;
public:
Matrix(int a = 0, int b = 0, int c = 0, int d = 0)
{
this->a = a;
this->b = b;
this->c = c;
this->d = d;
}
friend Matrix operator >> (Matrix op1, int arr[]);
friend Matrix &operator << (Matrix &op2, int arr[]);
void show()
{
cout << "Matrix = {" << a << ' ' << b << ' ' << c << ' ' << d << "} \n";
}
};
Matrix operator >> (Matrix op1, int arr[])
{
int* p = arr;
p[0] = op1.a;
p[1] = op1.b;
p[2] = op1.c;
p[3] = op1.d;
return *p;
}
Matrix& operator << (Matrix& op2, int arr[])
{
op2.a = arr[0];
op2.b = arr[1];
op2.c = arr[2];
op2.d = arr[3];
return op2;
}
int main()
{
Matrix a(4, 3, 2, 1), b;
int x[4], y[4] = { 1, 2, 3, 4 };
a >> x;
b << y;
for (int i = 0; i < 4; i++)
cout << x[i] << ' ';
cout << endl;
b.show();
}
8. 원을 추상화한 Circle 클래스는 간단히 아래와 같다. . . . 다음 연산이 가능하도록 연산자를 프렌드 함수로 작성하라
[결과]

[메인 함수]
#include <iostream>
#include <string>
using namespace std;
class Circle
{
int radius;
public:
Circle(int radius = 0) { this->radius = radius; }
friend Circle& operator++ (Circle &op);
friend Circle& operator++(Circle& op, int x);
void show() { cout << "radius = " << radius << " 인 원" << endl; }
};
Circle& operator++ (Circle& op)
{
++op.radius;
return op;
}
Circle& operator++ (Circle& op, int x)
{
Circle tmp = op;
op.radius++;
return tmp;
}
int main()
{
Circle a(5), b(4);
++a;
b = a++;
a.show();
b.show();
}
9. 문제 8번의 Circle 객체에 대해 다음 연산이 가능하도록 연산자를 구현하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class Circle
{
int radius;
public:
Circle(int radius = 0) { this->radius = radius; }
friend Circle operator + (int x, Circle op);
void show() { cout << "radius = " << radius << " 인 원" << endl; }
};
Circle operator + (int x, Circle op)
{
int y = x + op.radius;
return y;
}
int main()
{
Circle a(5), b(4);
b = 1 + a;
a.show();
b.show();
}
10. 통계를 내는 Statistic 클래스를 만들려고 한다. 데이터는 Statistic 클래스 내부에 int 배열을 동적으로 할당받아 유지한다. 다음과 같은 연산이 잘 이루어지도록 Statistic 클래스와 ! , >> , << , ~ 연산자 함수를 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class Statistic
{
int i = 0;
int count = 0;
int* pArray = new int[50];
bool isClear;
public:
Statistic()
{
for (int i = 0; i < 50; i++)
pArray[i] = 0;
isClear = true;
}
~Statistic()
{
delete[] pArray;
}
bool operator ! ()
{
if (isClear)
return true;
else
return false;
}
void operator ~()
{
for (int i = 0; i < count; i++)
{
cout << pArray[i] << ' ';
}
cout << endl;
}
Statistic& operator << (int x)
{
isClear = false;
pArray[i] = x;
i++;
count++;
return *this;
}
void operator >> (int &avg)
{
int sum = 0;
for (int i = 0; i < count; i++)
{
sum += pArray[i];
}
avg = sum / count;
}
};
int main()
{
Statistic stat;
if (!stat) cout << "현재 통계 데이터가 없습니다." << endl;
int x[5];
cout << "5개의 정수를 입력하라 >> ";
for (int i = 0; i < 5; i++)
cin >> x[i];
for (int i = 0; i < 5; i++)
stat << x[i];
stat << 100 << 200;
~stat;
int avg;
stat >> avg;
cout << "avg = " << avg << endl;
}
11. 스택 클래스 Stack을 만들고 푸시(push)용으로 << 연산자를, 팝(pop)을 위해 >> 연산자를, 비어 있는 스택인자를 알기 위해 ! 연산자를 작성하라, 다음 코드를 main()으로 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class Stack
{
int size = 3, count = 2, i = 0;
int* pArray = new int[size];
public:
Stack()
{
for (int i = 0; i < size; i++)
pArray[i] = 0;
}
~Stack()
{
delete[]pArray;
}
Stack &operator << (int x)
{
pArray[i] = x;
i++;
return *this;
}
void operator >> (int &x)
{
x = pArray[count];
count--;
size--;
}
bool operator ! ()
{
if (size == 0)
return true;
else
return false;
}
};
int main()
{
Stack stack;
stack << 3 << 5 << 10;
while (true)
{
if (!stack)
break;
int x;
stack >> x;
cout << x << ' ';
}
cout << endl;
}
12. 정수 배열을 항상 증가 순으로 유지하는 SortedArray 클래스를 작성하려고 한다. 아래의 main() 함수가 작동할 만큼만 SortedArray 클래스를 작성하고 +와 = 연산자도 작성하라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
class SortedArray {
int size; // 현재 배열의 크기
int* p; // 정수 배열에 대한 포인터
void sort(); // 정수 배열을 오름차순으로 정렬
public:
SortedArray(); // p는 NULL로 size는 0으로 초기화
SortedArray(SortedArray& src); // 복사 생성자
SortedArray(int p[], int size); // 생성자. 정수 배열과 크기를 전달받음
~SortedArray(); // 소멸자
SortedArray operator+ (SortedArray& op2); // 현재 배열에 op2 배열 추가
SortedArray& operator= (const SortedArray& op2); // 현재 배열에 op2 배열 복사
void show(); // 배열의 원소 출력
};
void SortedArray::show() {
sort();
cout << "배열 출력 : ";
for (int i = 0; i < size; i++)
cout << p[i] << ' ';
cout << endl;
}
SortedArray::SortedArray() {
size = 0;
p = NULL;
}
SortedArray::SortedArray(int p[], int size) {
this->p = new int[size];
this->size = size;
for (int i = 0; i < size; i++)
this->p[i] = p[i];
}
SortedArray::SortedArray(SortedArray& src) {
this->size = src.size;
this->p = new int[this->size];
for (int i = 0; i < this->size; i++)
this->p[i] = src.p[i];
}
SortedArray& SortedArray::operator= (const SortedArray& op2) {
delete[] p; // 배열 메모리 delete
this->size = op2.size;
this->p = new int[this->size]; // 객체 op2의 크기만큼 다시 동적 할당
for (int i = 0; i < this->size; i++)
this->p[i] = op2.p[i];
return *this;
}
SortedArray SortedArray::operator+ (SortedArray& op2) {
SortedArray test;
test.size = this->size + op2.size;
test.p = new int[test.size];
for (int i = 0; i < test.size; i++) {
if (i < this->size)
test.p[i] = this->p[i];
else
test.p[i] = op2.p[i - (test.size - op2.size)]; //total size - op2.size = this->size
}
return test;
}
void SortedArray::sort() {
int swp;
for (int i = 0; i < size; i++) { //p[0] ~ p[size] sequential sort
for (int j = i; j < size; j++) {
if (p[i] < p[j] || p[i] == p[j]);
else {
swp = p[i];
p[i] = p[j];
p[j] = swp;
}
}
}
}
SortedArray::~SortedArray() {
if (p) delete[] p;
}
int main() {
int n[] = { 2, 20, 6 };
int m[] = { 10, 7, 8, 30 };
SortedArray a(n, 3), b(m, 4), c;
c = a + b; // +, = 연산자 작성 필요
// + 연산자가 SortedArray 객체를 리턴하므로 복사 생성자 필요
a.show();
b.show();
c.show();
}
책에서 나오는 힌트나 조건들의 일부는 저작자 존중을 위해 일부러 작성하지 않았습니다.
개인 공부용으로 올리는 만큼 풀이에 대한 지적 감사하겠습니다.
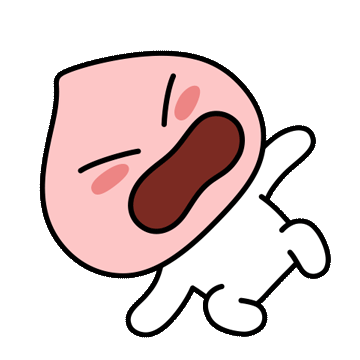
'C-C++ > 명품 C++ Programming' 카테고리의 다른 글
[(개정판) 명품 C++ programming] 9장 실습 문제 (0) | 2023.01.07 |
---|---|
[(개정판) 명품 C++ programming] 8장 실습 문제 (2) | 2023.01.07 |
[(개정판) 명품 C++ programming] 6장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 5장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 4장 실습 문제 (2) | 2023.01.05 |