1. add() 함수를 호출하는 main()함수는 다음과 같다. . .
1-1. add() 함수를 중복 작성하고 프로그램을 완성하라.
[소스 코드]
#include<iostream>
using namespace std;
int add(int x[], int y)
{
int sum = 0;
for (int i = 0; i < y; i++)
{
sum += x[i];
}
return sum;
}
int add(int x[], int y, int z[])
{
int sum = 0;
for (int i = 0; i < y; i++)
{
sum += x[i] + z[i];
}
return sum;
}
int main()
{
int a[] = { 1, 2, 3, 4, 5 };
int b[] = { 6, 7, 8, 9, 10 };
int c = add(a, 5);
int d = add(a, 5, b);
cout << c << endl;
cout << d << endl;
}
1-2. 디폴트 매개 변수를 가진 하나의 add() 함수를 작성하고 프로그램을 완성하라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
int add(int x[], int y = 5)
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum += x[i];
}
return sum;
}
int add(int x[], int y[], int z = 5)
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum += x[i] + y[i];
}
return sum;
}
int main()
{
int a[] = { 1, 2, 3, 4, 5 };
int b[] = { 6, 7, 8, 9, 10 };
int c = add(a);
int d = add(a, b);
cout << c << endl;
cout << d << endl;
}
2. Person 클래스의 객체를 생성하는 main() 함수는 다음과 같다. . . .
2-1. 생성자를 중복 작성하고, 프로그램을 완성하라.
[소스 코드]
#include<iostream>
using namespace std;
int add(int x[], int y = 5)
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum += x[i];
}
return sum;
}
int add(int x[], int y[], int z = 5)
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum += x[i] + y[i];
}
return sum;
}
int main()
{
int a[] = { 1, 2, 3, 4, 5 };
int b[] = { 6, 7, 8, 9, 10 };
int c = add(a);
int d = add(a, b);
cout << c << endl;
cout << d << endl;
}
2-2 디폴트 매개 변수를 가진 하나의 생성자를 작성하고 프로그램을 완성하라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
class Person
{
int id;
double weight = 20.5;
string name;
public:
Person(int id = 1, string name = "Grace", double weight = 20.5) { this->id = id; this->name = name; this->weight = weight; }
void show() { cout << id << ' ' << weight << ' ' << name << endl; }
};
int main()
{
Person grace, ashley(2, "Ashley"), helen(3, "Hellen", 32.5);
grace.show();
ashley.show();
helen.show();
}
3. 함수 big()을 호출하는 경우는 다음과 같다. . . .
3-1. big() 함수를 2개 중복하여 작성하고 프로그램을 완성하라.
[소스 코드]
#include<iostream>
using namespace std;
int big(int x, int y)
{
int max = 100;
int bigger = x;
if (x < y)
bigger = y;
if (bigger > max)
return max;
else if (bigger < max)
return bigger;
}
int big(int x, int y, int z)
{
int max = z;
int bigger = x;
if (x < y)
bigger = y;
if (bigger > max)
return max;
else if (bigger < max)
return bigger;
}
int main()
{
int x = big(3, 5);
int y = big(300, 60);
int z = big(30, 60, 50);
cout << x << ' ' << y << ' ' << z << endl;
}
3-2. 디폴트 매개 변수를 가진 하나의 함수로 big()을 작성하고 프로그램을 완성하라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
int big(int x, int y, int z = 100)
{
int max = z;
int bigger = x;
if (x < y)
bigger = y;
if (bigger > max)
return max;
else if (bigger < max)
return bigger;
}
int main()
{
int x = big(3, 5);
int y = big(300, 60);
int z = big(30, 60, 50);
cout << x << ' ' << y << ' ' << z << endl;
}
4. 다음 클래스에 중복된 생성자를 디폴트 매개 변수를 가진 하나의 생성자로 작성하고 테스트 프로그램을 작성하라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
class MyVector
{
int* mem;
int size;
public:
MyVector(int n = 100, int val = 0);
~MyVector() { delete[]mem; }
void show();
};
MyVector:: MyVector(int n, int val)
{
mem = new int[n];
size = n;
for (int i = 0; i < size; i++)
mem[i] = val;
}
void MyVector::show()
{
for (int i = 0; i < size; i++)
cout << mem[i] << ' ';
cout << endl;
}
int main()
{
int x, y;
x = 200, y = 5;
MyVector Vector1;
MyVector Vector2(x, y);
Vector1.show();
Vector2.show();
}
5. 동일한 크기로 배열을 변환하는 다음 2개의 static 멤버 함수를 가진 ArrayUtility 클래스를 만들어라.
[결과]

[소스 코드]
#include<iostream>
using namespace std;
class ArrayUtility
{
public:
ArrayUtility() {}
static void intToDouble(int source[], double dest[], int size)
{
for (int i = 0; i < size; i++)
{
dest[i] = source[i];
}
}
static void doubleToInt(double source[], int dest[], int size)
{
for (int i = 0; i < size; i++)
{
dest[i] = source[i];
}
}
};
int main()
{
int x[] = { 1, 2, 3, 4, 5 };
double y[5];
double z[] = { 9.9, 8.8, 7.7, 6.6, 5.6 };
ArrayUtility::intToDouble(x, y, 5);
for (int i = 0; i < 5; i++)
cout << y[i] << ' ';
cout << endl;
ArrayUtility::doubleToInt(z, x, 5);
for (int i = 0; i < 5; i++)
cout << x[i] << ' ';
cout << endl;
}
6. 동일한 크기의 배열을 변환하는 다음 2개의 static 멤버 함수를 가진 ArrayUtility2 클래스를 만들고, 이 클래스를 이용하여 아리 결과와 같이 출력되도록 프로그램을 완성하라.
[결과]

[소스 코드]
class ArrayUtility2{
public:
// s1과 s2를 연결한 새로운 배열을 동적 생성하고 포인터 리턴
static int* concat(int s1[], int s2[], int size);
// s1에서 s2에 있는 숫자를 모두 삭제한 새로운 배열을 동적 생성하여 리턴
// 리턴하는 배열의 크기는 retSize에 전달. retSize가 0인 경우 NULL 리턴
static int* remove(int s1[], int s2[], int size, int& retSize);
};
int* ArrayUtility2::concat(int s1[], int s2[], int size){
int* tot = new int [size]; // 새로운 배열 동적 생성
for(int i=0; i<size/2; i++) {
tot[i] = s1[i];
}
for(int i=size/2, j=0; i<size; i++,j++){
tot[i] = s2[j];
}
return tot;
}
int* ArrayUtility2::remove(int s1[], int s2[], int size, int& retSize){
int* rmtot = new int [size/2];
int cnt;
for(int i=0; i<size/2; i++){
cnt = 0;
for(int j=0; j<size/2; j++){
if(s1[i] == s2[j]) {
cnt++;
}
}
if(cnt == 0) {
rmtot[i] = s1[i];
retSize++;
}
}
if(retSize == 0) return NULL;
return rmtot;
}
int main() {
int x[5], y[5];
int size,retSize = 0;
int *p;
cout << "정수 5 개 입력하라. 배열 x에 삽입한다>>";
for(int i=0; i<5; i++){
cin >> x[i];
}
cout << "정수 5 개 입력하라. 배열 y에 삽입한다>>";
for(int i=0; i<5; i++){
cin >> y[i];
}
size = (sizeof(x) + sizeof(y)) / sizeof(int);
cout << "합친 정수 배열을 출력한다\n";
p = ArrayUtility2::concat(x,y,size);
for(int i=0; i<size; i++){
cout << p[i] << ' ';
}
cout << endl << "배열 x[]에서 y[]를 뺀 결과를 출력한다. 개수는 ";
p = ArrayUtility2::remove(x,y,size,retSize);
cout << retSize << endl;
for(int i=0; i<retSize; i++){
cout << p[i] << ' ';
}
}
7. 다음과 같은 static 멤버를 가진 Random 클래스를 완성하라. 그리고 Random 쿨래스를 이용하여 다음과 같이 랜덤한 값을 출력하는 main() 함수도 작성하라. main() 에서 Random 클래스의 seed() 함수를 활용하라.
[결과]

[소스 코드]
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
class Random
{
public:
static void seed() { srand((unsigned)time(0)); }
static int nextInt(int min = 0, int max = 32767)
{
int n = (rand() % (max - min + 1)) + min;
return n;
}
static char nextAlphabet()
{
int n = (rand() % 26) + 65;
char alphabet = (char)n;
return alphabet;
}
static double nextDouble()
{
double N = 32767;
double n = rand() / N;
return n;
}
};
int main()
{
cout << "1에서 100까지의 랜덤한 정수 10개를 출력합니다. " << endl;
Random::seed();
for (int i = 0; i < 10; i++)
{
cout << Random::nextInt(1, 100) << ' ';
}
cout << endl;
cout << "알파벳을 랜덤하게 10개 출력합니다." << endl;
for (int i = 0; i < 10; i++)
{
cout << Random::nextAlphabet() << ' ';
}
cout << endl;
cout << "랜덤한 실수를 10개를 출력합니다." << endl;
for (int i = 0; i < 10; i++)
{
cout << Random::nextDouble() << ' ';
if (i == 4)
cout << endl;
}
cout << endl;
}
8. 디버깅에 필요한 정보를 저장하는 Trace 클래스를 만들어보자. 저자의 경험에 의하면, 멀티테스크 프로그램을 개발하거나 특별한 환경에서 작업할 때, Visual Studio의 디버거와 같은 소스 레벨 디버거를 사용하지 못하는 경우가 더러 있었고, 이때 실행 도중 정보를 저장하기 위해 Trace 클래스를 만들어 사용하였다. Trace클래스를 활용하는 다음 프로그램과 결과를 참고하여 Trace 클래스를 작성하고 전체 프로그램을 완성하라. 디버깅 정보는 100개로 제한한다.
[결과]

[소스 코드]
#include<iostream>
#include<string>
using namespace std;
class Trace
{
public:
static string tagInf[100];
static string debugInf[100];
static int count;
static void put(string tag, string debug);
static void print(string tag = "all");
};
int Trace::count = 0;
string Trace::tagInf[100];
string Trace::debugInf[100];
void f()
{
int a, b, c;
cout << "두 개의 정수를 입력하세요>>";
cin >> a >> b;
Trace::put("f()", "정수를 입력 받았음");
c = a + b;
Trace::put("f()", "합 계산");
cout << "합은 " << c << endl;
}
void Trace::put(string tag, string debug)
{
tagInf[count] = tag;
debugInf[count] = debug;
count++;
}
void Trace::print(string tag)
{
if (tag == "all")
{
cout << "----- 모든 Trace 정보를 출력합니다. -----\n";
for (int i = 0; i < count; i++)
{
cout << tagInf[i] << ":" << debugInf[i] << endl;
}
}
else
{
cout << "----- " << tag << "태그의 Trace 정보를 출력합니다. -----\n";
for (int i = 0; i < count; i++)
{
if (tagInf[i] == tag) cout << tagInf[i] << ":" << debugInf[i] << endl;
}
}
}
int main()
{
Trace::put("main()", "프로그램을 시작합니다");
f();
Trace::put("main()", "종료");
Trace::print("f()");
Trace::print();
}
9. 게시판 프로그램을 작성해보자. 멀티테스킹의 경우 여러 사용자들이 게시판에 글을 올리기 때문에 게시판 객체는 전체 하나만 있어야 한다. 그러므로 게시판 객체의 멤버들은 static으로 작성한다. 다음은 게시판 기능을 하는 Board 클래스를 활용하는 main() 코드이다. 실행 결과를 참고하여 Board 클래스를 만들고 전체 프로그램을 완성하라. static 연습이 목적이기 때문에 게시판 기능을 글을 올리는 기능과 게시글을 모두 출력하는 기능으로 제한하고 main()도 단순화하였다.
[결과]

[소스 코드]
#include<iostream>
#include<string>
using namespace std;
class Board
{
static int count;
static string strList [100];
public:
static void add(string str);
static void print();
};
int Board::count = 0;
string Board::strList[100];
void Board::add(string str)
{
strList[count] = str;
count++;
}
void Board::print()
{
cout << "*********** 게시판입니다. *************" << endl;
for (int i = 0; i < count; i++)
{
cout << i << " : " << strList[i] << endl;
}
}
int main()
{
Board::add("중간고사는 감독 없는 자율 시험입니다.");
Board::add("코딩 라운지 많이 이용해 주세요");
Board::print();
Board::add("진소린 학생이 경진대회 입상하였습니다. 축하해주세요");
Board::print();
}
책에서 나오는 힌트나 조건들의 일부는 저작자 존중을 위해 일부러 작성하지 않았습니다.
개인 공부용으로 올리는 만큼 풀이에 대한 지적 감사하겠습니다.
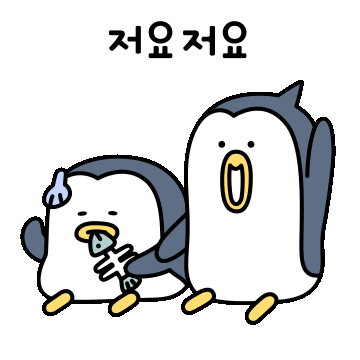
'C-C++ > 명품 C++ Programming' 카테고리의 다른 글
[(개정판) 명품 C++ programming] 8장 실습 문제 (2) | 2023.01.07 |
---|---|
[(개정판) 명품 C++ programming] 7장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 5장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 4장 실습 문제 (2) | 2023.01.05 |
[(개정판) 명품 C++ programming] 3장 실습 문제 (0) | 2023.01.05 |