1. main()의 실행 결과가 다음과 같도록 Tower 클래스를 작성하여라.
[main()]
#include <iostream>
using namespace std;
int main()
{
Tower myTower;
Tower seoulTower(100);
cout << "높이는 " << myTower.getHeight() << "미터" << endl;
cout << "높이는 " << seoulTower.getHeight() << "미터" << endl;
}
[결과]

[소스 코드]
#include <iostream>
using namespace std;
class Tower
{
int height;
public:
Tower() { height = 1; }
Tower(int height) { this->height = height; }
int getHeight() { return height; }
};
int main()
{
Tower myTower;
Tower seoulTower(100);
cout << "높이는 " << myTower.getHeight() << "미터" << endl;
cout << "높이는 " << seoulTower.getHeight() << "미터" << endl;
}
2. 날짜를 다루는 Date 클래스를 작성하고자 한다. Date를 이용하는 main()과 실행 결과는 다음과 같다. 클래스 Date를 작성하여 아래 프로그램에 추가하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class Date {
int year;
int month;
int day;
public:
Date(int year, int month, int day)
{
this->year = year;
this->month = month;
this->day = day;
}
Date(string date);
void show() { cout << year << "년" << month << "월" << day << "일" << endl; }
int getYear() { return year; }
int getMonth() { return month; }
int getDay() { return day; }
};
Date::Date(string date)
{
int index;
this->year = stoi(date);
index = date.find('/');
this->month = stoi(date.substr(index + 1));
index = date.find('/', index + 1);
this->day = stoi(date.substr(index + 1));
}
int main() {
Date birth(2014, 3, 20);
Date independenceDay("1945/8/15");
independenceDay.show();
cout << birth.getYear() << ',' << birth.getMonth() << ',' << birth.getDay() << endl;
}
3. 은행에서 사용하는 프로그램을 작성하기 위해, 은행 계좌 하나를 표현하는 클래스 Account가 필요하다. 계좌 정보는 계좌 주인, 계좌 번호, 잔액을 나타내는 3 멤버 변수로 이루어진다. main()함수의 실행 결과가 다음과 같도록 Account 클래스를 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class Account
{
int id, balance = 0;
string name;
public:
Account(string name, int id, int balance) { this->name = name; this->id = id; this->balance = balance; }
string getOwner() { return name; }
int deposit(int balance) { this->balance += balance; return this->balance; }
int inquiry() { return balance; }
int withdraw(int balance) { this->balance -= balance; return this->balance; }
};
int main()
{
Account a("Kitae", 1, 5000);
a.deposit(50000);
cout << a.getOwner() << "의 잔액은 " << a.inquiry() << endl;
int money = a.withdraw(20000);
cout << a.getOwner() << "의 잔액은 " << a.inquiry() << endl;
}
4. CoffeeMachine 클래스를 만들어보자. main() 함수와 실행하는 결과가 다음과 같도록 CoffeeMachine 클래스를 작성해라. 에스프레소 한 잔에는 커피와 물이 각각 1씩 소비되고, 아메리카노의 경우 커피는 1, 물은 2가 소비되고, 설탕 커피는 커피 1, 물 2, 설탕 1이 소모된다. CoffeeMachine 클래스에는 어떤 멤버 변수와 어떤 멤버 함수가 필요한지 잘 판단하여 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <string>
using namespace std;
class CoffeeMachine
{
int coffee, water, sugar;
public:
CoffeeMachine(int coffee, int water, int sugar) { this->coffee = coffee; this->water = water; this->sugar = sugar; }
void show();
int drinkEspresso();
int drinkAmericano();
int drinkSugarCoffee();
void fill() { coffee = 10; water = 10; sugar = 10; }
};
void CoffeeMachine::show()
{
cout << "커피머신 상태 > " << "커피 : " << coffee << ' ' << "물 : " << water << ' ' <<"설탕 : " << sugar << endl;
}
int CoffeeMachine::drinkAmericano()
{
coffee = coffee - 1;
water = water - 2;
return coffee, water;
}
int CoffeeMachine::drinkEspresso()
{
coffee = coffee - 1;
water = water - 1;
return coffee, water;
}
int CoffeeMachine::drinkSugarCoffee()
{
coffee = coffee - 1;
water = water - 2;
sugar = sugar - 1;
return coffee, water, sugar;
}
int main()
{
CoffeeMachine java(5, 10, 3);
java.drinkEspresso();
java.show();
java.drinkAmericano();
java.show();
java.drinkSugarCoffee();
java.show();
java.fill();
java.show();
}
5. 랜덤 수를 발생시키는 Random 클래스를 만들자. Random 클래스를 이용하여 랜덤 한 정수 10개를 출력하는 사례는 다음과 같다. Random 클래스가 생성자, next(), nextInRange()의 세개의 멤버 함수를 가지도록 작성하고 main()함수와 합쳐 하나의 ccp 파일에 구현하라.
[결과]

[소스 코드]
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Random
{
int randomInt;
public:
int next();
int nextInRange(int a, int b);
};
int Random::next()
{
int n = rand();
return n;
}
int Random::nextInRange(int a, int b)
{
int n = rand() % (b -a + 1) + a;
return n;
}
int main()
{
Random r;
cout << "-- 0에서 " << RAND_MAX << "까지의 랜덤 정수 10개 --" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.next();
cout << n << ' ';
}
cout << endl << endl << "-- 2에서 " << "4까지의 랜덤 정수 10개 --" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.nextInRange(2, 4);
cout << n << ' ';
}
cout << endl;
}
6. 문제 5번을 참고하여 짝수 정무산 랜덤하게 발생시키는 EvenRandom 클래스를 작성하고 EvenRandom 클래스를 이용하여 10개의 짝수를 랜덤하게 출력하는 프로그램을 완성하라. 0도 짝수로 처리한다.
[결과]

[소스 코드]
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Random
{
public:
int next();
int nextInRange(int a, int b);
};
int Random::next()
{
while (true)
{
int n = rand();
if (n % 2 == 0)
{
return n;
}
}
}
int Random::nextInRange(int a, int b)
{
while (true)
{
int n = rand() % (b - 1) + a;
if (n % 2 == 0)
{
return n;
}
}
}
int main()
{
Random r;
cout << "-- 0에서 " << RAND_MAX << "까지의 랜덤 정수 10개 --" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.next();
cout << n << ' ';
}
cout << endl << endl << "-- 2에서 " << "4까지의 랜덤 정수 10개 --" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.nextInRange(2, 10);
cout << n << ' ';
}
cout << endl;
}
7. 문제 5번을 참고하여 생성자를 이용하여 짝수 홀수를 선택할 수 있도록 Selectable Random 클래스를 작성하고 짝수 10개, 홀수 10개를 랜덤하게 발생시키는 프로그램을 작성하라.
[결과]

[소스 코드]
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Random
{
string first, second;
public:
int next();
int nextInRange(int a, int b);
void inputKind(string first, string second) { this->first = first; this->second = second; }
};
int Random::next()
{
while (true)
{
int n = rand();
if (first == "짝수")
{
if (n % 2 == 0)
{
return n;
}
}
else if (first == "홀수")
{
if (n % 2 == 1)
{
return n;
}
}
}
}
int Random::nextInRange(int a, int b)
{
while (true)
{
int n = rand() % (b - 1) + a;
if (second == "짝수")
{
if (n % 2 == 0)
{
return n;
}
}
else if (second == "홀수")
{
if (n % 2 == 1)
{
return n;
}
}
}
}
int main()
{
string first, second;
Random r;
cout << "1번과 2번의 종류를 각각 정해주세요 >> ";
cin >> first >> second;
r.inputKind(first, second);
cout << "-- 0에서 " << RAND_MAX << "까지의 랜덤 " << first << " 정수 10개--" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.next();
cout << n << ' ';
}
cout << endl << endl << "-- 2에서 " << "4까지의 랜덤 " << second << " 정수 10개--" << endl;
for (int i = 0; i < 10; i++)
{
int n = r.nextInRange(2, 10);
cout << n << ' ';
}
cout << endl;
}
8. int 타입의 정수를 객체화한 Integer 클래스를 작성하라. Integer의 모든 멤버 함수를 자동 인라인으로 작성하라. Integer 클래스를 활용하는 코드는 다음과 같다.
[결과]

[소스 코드]
#include <iostream>
#include <cstdlib>
#include <string>
using namespace std;
class Integer
{
int num;
public:
Integer(int num) { this->num = num; }
Integer(string nums) { num = stoi(nums); }
int get() { return num; }
void set(int num) { this->num = num; }
bool isEven() { return true; }
};
int main()
{
Integer n(30);
cout << n.get() << ' ';
n.set(50);
cout << n.get() << ' ';
Integer m("300");
cout << m.get() << ' ';
cout << m.isEven();
cout << endl;
}
9. Oval 클래스는 주어진 사각형에 내접하는 타원을 추상화한 클래스이다. Oval 클래스의 멤버는 모두 다음과 같다. Oval 클래스를 선언부와 구현부로 나누어 작성하라.
[결과]

[선언부 Oval.h]
#ifndef OVAL_H
#define OVAL_H
class Oval
{
int width, height;
public:
Oval() { width = 1; height = 1; }
Oval(int width, int height);
void set(int width, int height);
void show();
int getWidth();
int getHeight();
~Oval();
};
#endif
[구현부 Oval.cpp]
#include <iostream>
#include "Oval.h"
using namespace std;
Oval::Oval(int width, int height)
{
this->width = width;
this->height = height;
}
void Oval::set(int width, int height)
{
this->width = width;
this->height = height;
}
void Oval::show()
{
cout << "Width = " << width << " " << "Height = " << height << endl;
}
int Oval::getWidth()
{
return width;
}
int Oval::getHeight()
{
return height;
}
Oval::~Oval()
{
{ cout << "Oval 소멸 : width = " << width << " height = " << height << endl; }
}
[메인 함수]
#include <iostream>
#include <cstdlib>
#include <string>
#include "Oval.h"
using namespace std;
int main()
{
Oval a, b(3, 4);
a.set(10, 20);
a.show();
cout << b.getWidth() << "," << b.getHeight() << endl;
}
10. 다수의 클래스를 선언하고 활용하는 간단한 문제이다. 더하기(+), 빼기(-), 곱하기(*), 나누기(/)를 수행하는 4개의 클래스 Add, Sub, Mul, Div를 만들고자 한다. . . .(부가 설명 생략)
[결과]

[선언부 Add.h]
#ifndef ADD_H
#define ADD_H
class Add
{
int x, y;
public:
void setValue(int x, int y);
int calculate();
};
#endif
//Sub, Mul, Div 모두 클래스 명 외에는 모두 같다.
[구현부]
#include <iostream>
#include "Add.h"
#include "Sub.h"
#include "Mul.h"
#include "Div.h"
using namespace std;
void Add::setValue(int x, int y)
{
this->x = x;
this->y = y;
}
int Add :: calculate()
{
return x + y;
}
void Sub::setValue(int x, int y)
{
this->x = x;
this->y = y;
}
int Sub::calculate()
{
return x - y;
}
void Mul::setValue(int x, int y)
{
this->x = x;
this->y = y;
}
int Mul::calculate()
{
return x * y;
}
void Div::setValue(int x, int y)
{
this->x = x;
this->y = y;
}
int Div::calculate()
{
return x / y;
}
[메인 함수]
#include <iostream>
#include "Add.h"
#include "Sub.h"
#include "Mul.h"
#include "Div.h"
using namespace std;
int main()
{
int x, y;
char op;
Add a;
Sub s;
Mul m;
Div d;
while (true)
{
cout << "두 수와 연산자를 순서대로 입력해 주세요 >> ";
cin >> x >> y >> op;
if (op == '+')
{
a.setValue(x, y);
cout << a.calculate() << endl;
}
else if (op == '-')
{
s.setValue(x, y);
cout << s.calculate() << endl;
}
else if (op == '*')
{
m.setValue(x, y);
cout << m.calculate() << endl;
}
else if (op == '/')
{
d.setValue(x, y);
cout << d.calculate() << endl;
}
}
}
11. 다음 코드에서 Box 클래스의 선언부와 구현부를 Box.h, Box.cpp 파일로 분리하고, main() 함수 부분을 main.cpp로 분리하여 전체 프로그램을 완성하라.
[결과]

[선언부 Box.h]
#ifndef BOX_H
#define BOX_H
class Box
{
int width, height;
char fill;
public:
Box(int width, int height);
void setFill(char f);
void setSize(int w, int h);
void draw();
};
#endif
[구현부 Box.cpp]
#include <iostream>
#include "Box.h"
using namespace std;
Box::Box(int w, int h)
{
setSize(w, h);
fill = '*';
}
void Box::setFill(char f)
{
fill = f;
}
void Box::setSize(int w, int h)
{
width = w;
height = h;
}
void Box::draw()
{
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
cout << fill;
}
cout << endl;
}
}
#include <iostream>
#include "Box.h"
using namespace std;
int main()
{
Box b(10, 2);
b.draw();
cout << endl;
b.setSize(7, 4);
b.setFill('^');
b.draw();
}
12. 컴퓨터의 주기억장치를 모델링하는 클래스 Ram을 구현하ㅕ고 한다. Ram 클래스는 데이터가 기록될 메모리 공간과 크기 정보를 가지고, 주어진 주소에 데이터를 기록하고 (write). 주어진 주소로부터 데이터를 읽어온다. (read). Ram 클래스는 다음과 같이 선언한다.
[결과]

[선언부 Ram.h]
#ifndef RAM_H
#define RAM_H
class Ram
{
char mem[100 * 1024];
int size;
public:
Ram();
~Ram();
char read(int address);
void write(int address, char value);
};
#endif
[구현부 Ram.cpp]
#include <iostream>
#include "Ram.h"
using namespace std;
Ram::Ram()
{
for (int x = 0; x < 100; x++)
{
for (int y = 0; y < 1024; y++)
{
mem[x * y] = 0;
}
}
size = 100 * 1024;
}
Ram :: ~Ram()
{
cout << endl << "메모리 제거됨" << endl;
}
void Ram::write(int address, char value)
{
mem[address] = value;
}
char Ram::read(int address)
{
return mem[address];
}
[메인 함수]
#include <iostream>
#include "Ram.h"
using namespace std;
int main()
{
Ram ram;
ram.write(100, 20);
ram.write(101, 30);
char res = ram.read(100) + ram.read(101);
ram.write(102, res);
cout << "102 번지 값 = " << (int)ram.read(102);
}
책에서 나오는 힌트나 조건들의 일부는 저작자 존중을 위해 일부러 작성하지 않았습니다.
개인 공부용으로 올리는 만큼 풀이에 대한 지적 감사하겠습니다.
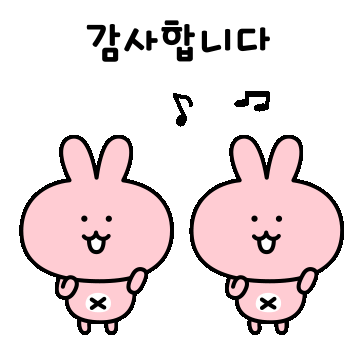
'C-C++ > 명품 C++ Programming' 카테고리의 다른 글
[(개정판) 명품 C++ programming] 7장 실습 문제 (0) | 2023.01.06 |
---|---|
[(개정판) 명품 C++ programming] 6장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 5장 실습 문제 (0) | 2023.01.06 |
[(개정판) 명품 C++ programming] 4장 실습 문제 (2) | 2023.01.05 |
[(개정판) 명품 C++ programming] 2장 실습 문제 (2) | 2023.01.04 |